魔方 (cube. cpp/c/pas)
题目描述
ccy (ndsf) 觉得手动复原魔方太慢了, 所以他要借助计算机.
ccy (ndsf) 家的魔方都是 333 的三阶魔方, 大家应该都见过.
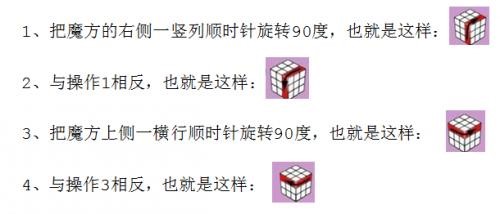
(3 的 “顺时针” 改为 “逆时针”, 即 3 4 以图为准.)
ccy (ndfs) 从网上搜了一篇攻略, 并找人翻译成了他自己会做的方法. 现在告诉你他的魔方情况, 以及他从网上搜到的攻略, 请你求出最后魔方变成什么样子.
输入格式
第一行, 一串数字, 表示从网上搜到的攻略.
下面 6*3 行, 每行 3 个数字, 每三行表示魔方一个面的情况, 六个面的顺序是前、后、左、右、上、下.
输出格式
6*3 行, 表示处理后的魔方, 形式同输入.
样例输入
23
121
221
111
123
321
111
123
321
132
132
231
132
121
112
233
332
111
333
样例输出
123
222
113
212
321
113
122
321
132
121
333
121
211
312
113
331
111
331
样例解释
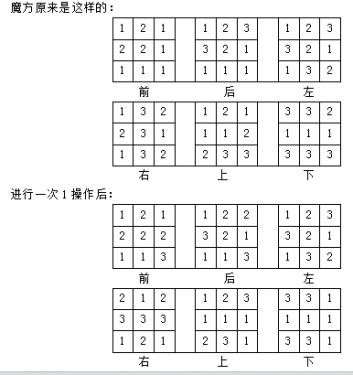
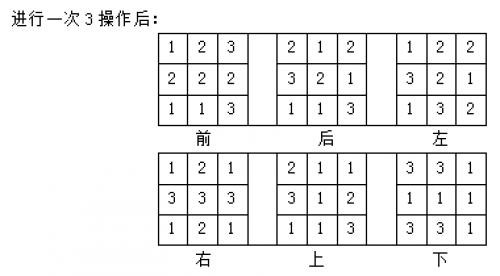
数据范围
40% 的数据, 攻略的长度小于 5 且仅有 4 种操作的其中一种
100% 的数据, 攻略的长度小于 100
Explanation
纯暴力+实现
Source Code
#include <iostream>
#include <cstdlib>
#include <cstdio>
#include <cstring>
using namespace std;
class RubikCube
{
public:
int face[6][3][3]; // Front, Rear, Left, Right, Top, Bottom
#define eax(_a,_b,_c) (this->face[_a-1][_b-1][_c-1])
void shift(int a1, int a2, int a3, int b1, int b2, int b3, int c1, int c2, int c3, int d1, int d2, int d3, bool cw) {
if (cw) { // Clockwise
int tmp = eax(d1,d2,d3);
eax(d1,d2,d3) = eax(c1,c2,c3);
eax(c1,c2,c3) = eax(b1,b2,b3);
eax(b1,b2,b3) = eax(a1,a2,a3);
eax(a1,a2,a3) = tmp;
} else { // Counterclockwise
int tmp = eax(a1,a2,a3);
eax(a1,a2,a3) = eax(b1,b2,b3);
eax(b1,b2,b3) = eax(c1,c2,c3);
eax(c1,c2,c3) = eax(d1,d2,d3);
eax(d1,d2,d3) = tmp;
}
return ;
}
void rot_1_2(bool cw) {
shift( 4,1,1, 4,1,3, 4,3,3, 4,3,1, cw);
shift( 4,1,2, 4,2,3, 4,3,2, 4,2,1, cw);
shift( 1,1,3, 5,1,3, 2,1,3, 6,1,3, cw);
shift( 1,2,3, 5,2,3, 2,2,3, 6,2,3, cw);
shift( 1,3,3, 5,3,3, 2,3,3, 6,3,3, cw);
}
void rot_3_4(bool cw) {
shift( 5,1,1, 5,1,3, 5,3,3, 5,3,1, cw);
shift( 5,1,2, 5,2,3, 5,3,2, 5,2,1, cw);
shift( 1,1,1, 4,1,1, 2,1,1, 3,1,1, cw);
shift( 1,1,2, 4,1,2, 2,1,2, 3,1,2, cw);
shift( 1,1,3, 4,1,3, 2,1,3, 3,1,3, cw);
}
} cube;
char str[200];
int main(int argc, char** argv)
{
scanf("%s", str);
for (int i = 1; i <= 6; i++)
for (int j = 1; j <= 3; j++)
for (int k = 1; k <= 3; k++) {
char c = getchar();
while (c < '0' || c > '9')
c = getchar();
cube.face[i-1][j-1][k-1] = c - '0';
}
for (int i = 0, len = strlen(str); i < len; i++) {
int method = str[i] - '0';
switch (method) {
case 1:
cube.rot_1_2(true);
break;
case 2:
cube.rot_1_2(false);
break;
case 3:
cube.rot_3_4(true);
break;
case 4:
cube.rot_3_4(false);
break;
default:
break;
}
}
// Done rotation, printing
for (int i = 1; i <= 6; i++)
for (int j = 1; j <= 3; j++) {
for (int k = 1; k <= 3; k++)
printf("%d", cube.face[i-1][j-1][k-1]);
printf("\n");
}
return 0;
}